Animation
基础用法
动画
和动画合集
通过自定义的动画id
执行所有的操作。 动画的操作包括:
- 创建动画
- 开始动画
- 暂停动画
- 恢复动画
- 取消动画
- 翻转动画
注册动画组件
<qt-animation
ref="animationRef"
class="animation-view-css">
<!-- 包裹需要执行动画的vue组件-->
<div class="animation-inner-view-class"/>
</qt-animation>
创建动画
创建单个动画和动画合集都需要自定义不重复的id
,用来后续执行动画的各种操作。
创建动画参数:
animationRef.value?.objectAnimator2( //创建2个属性值的动画
"1", //自定义id
QTAnimationValueType.QT_ANIMATION_VALUE_TYPE_FLOAT, //动画属性值类型
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_ALPHA, //动画属性类型
"0", //属性值
"1", //属性值
1000, //动画执行时间
-1, //动画重复执行模式
0, //动画重复执行次数
false, //是否监听动画执行状态变化
false, //是否监听动画执行属性值变化
{ //插值器参数对象
type: QTAnimationInterpolatorType.QT_ACCELERATE_INTERPOLATOR,
}
);
创建单个动画
创建2个参数的动画
animationRef.value?.objectAnimator2(参数)
创建3个参数的动画
animationRef.value?.objectAnimator3(参数)
创建4个参数的动画
animationRef.value?.objectAnimator4(参数)
创建5个参数的动画
animationRef.value?.objectAnimator5(参数)
以此类推,最多可以创建10个参数的动画。
示例代码
创建id为1
的2个参数动画
animationViewRef.value?.objectAnimator2(
"1",//自定义id
QTAnimationValueType.QT_ANIMATION_VALUE_TYPE_FLOAT,
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_ALPHA,
"0",
"1",
1000,
-1,
0,
false,
false,
null,
);
创建动画合集
animationViewRef.value?.animatorSet(参数)
示例代码
创建id为SequentiallyAnimatorsId
的动画合集
animationViewRef.value?.animatorSet('SequentiallyAnimatorsId', -1, false);
开始动画
参数为创建的动画的id
animationViewRef.value?.startAnimator("1");
延迟开始动画
参数为创建的动画的id
animationViewRef.value?.startAnimatorDelay("1", 2000);
暂停动画
参数为创建的动画的id
animationViewRef.value?.pauseAnimator("1");
恢复动画
参数为创建的动画的id
animationViewRef.value?.resumeAnimator("1");
取消动画
参数为创建的动画的id
animationViewRef.value?.cancelAnimator("1");
翻转动画
参数为创建的动画的id
animationViewRef.value?.reverseAnimator("1");
重置所有动画
animationViewRef.value?.resetAnimators();
动画属性
动画属性类型:alpha
效果预览
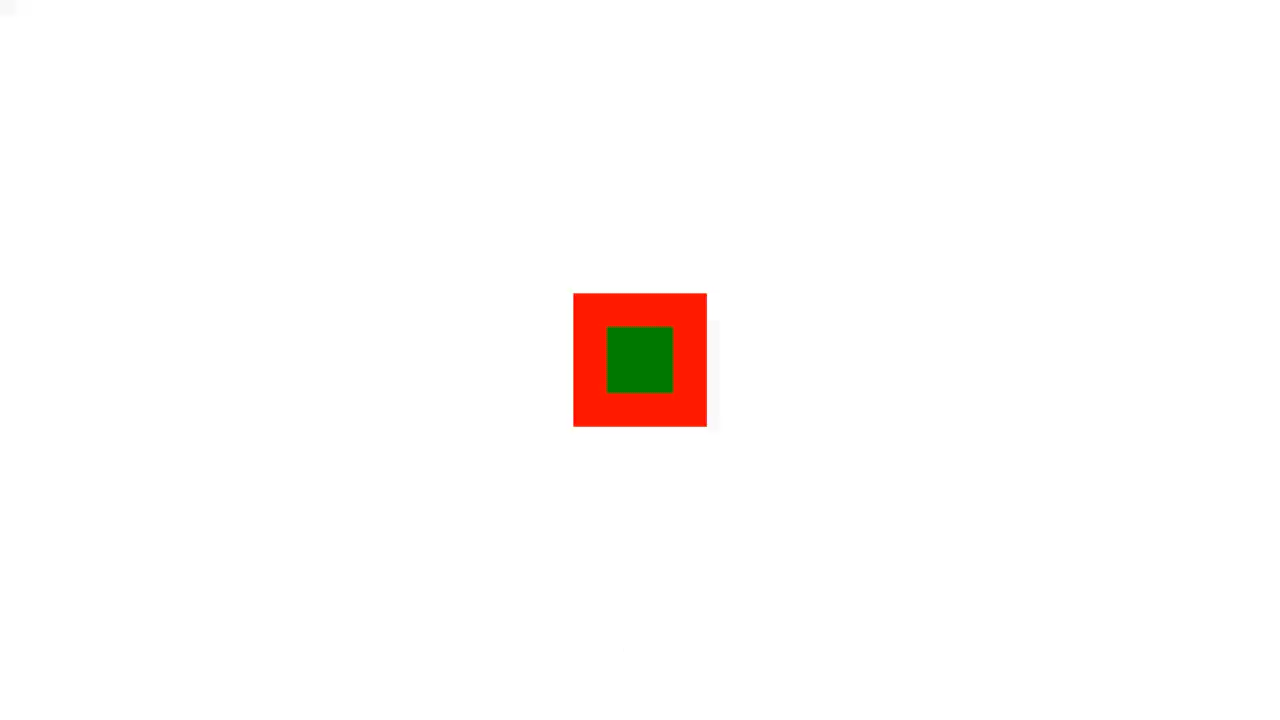
动画属性类型:rotation
效果预览
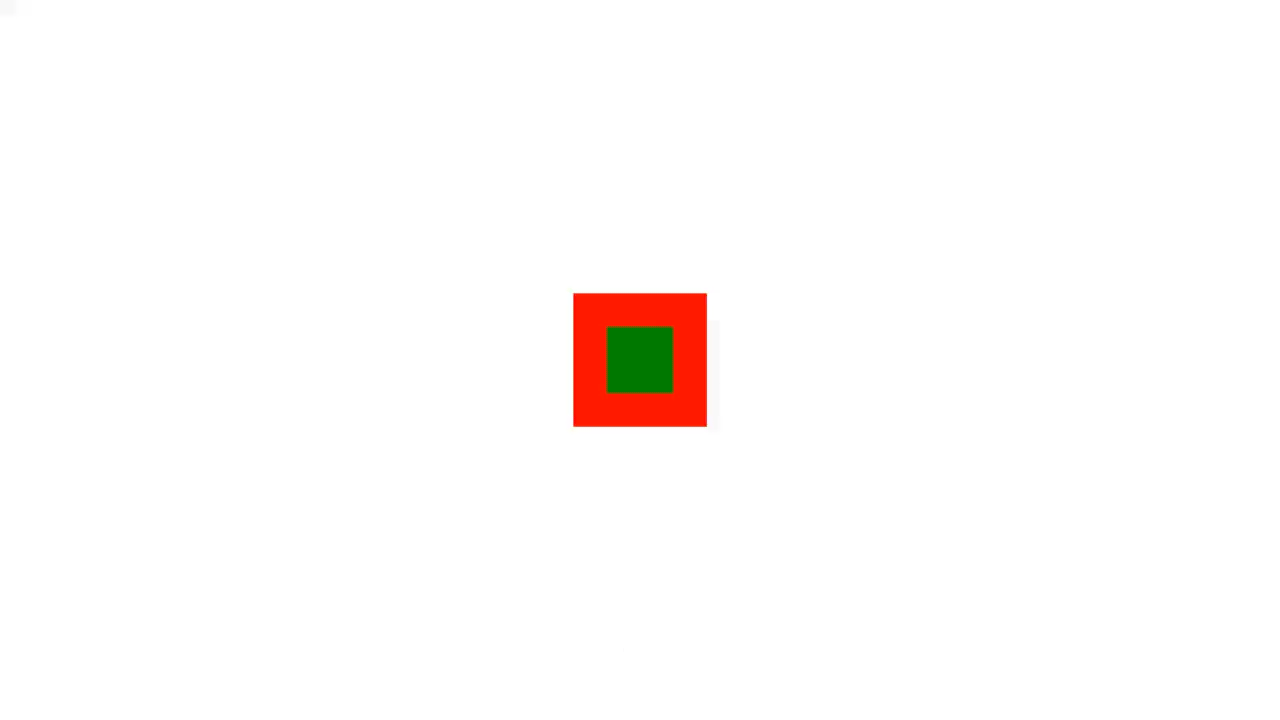
动画属性类型:scale
效果预览
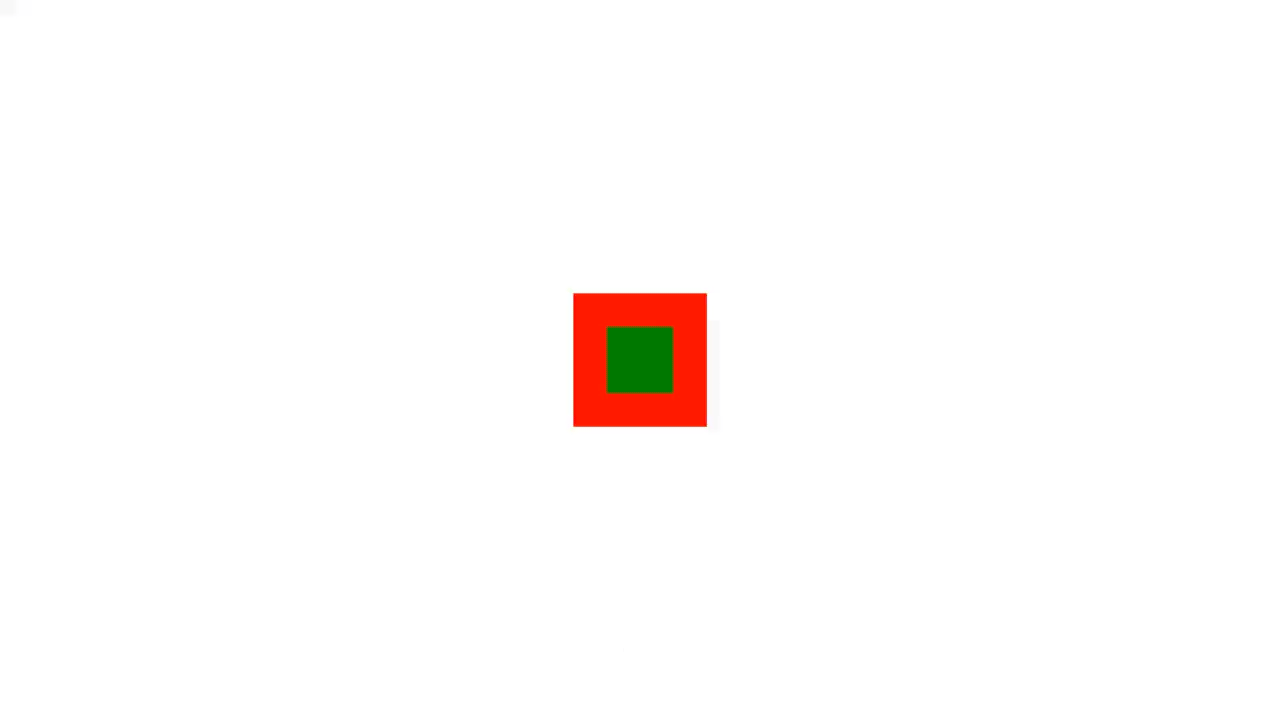
动画属性类型:translation
效果预览
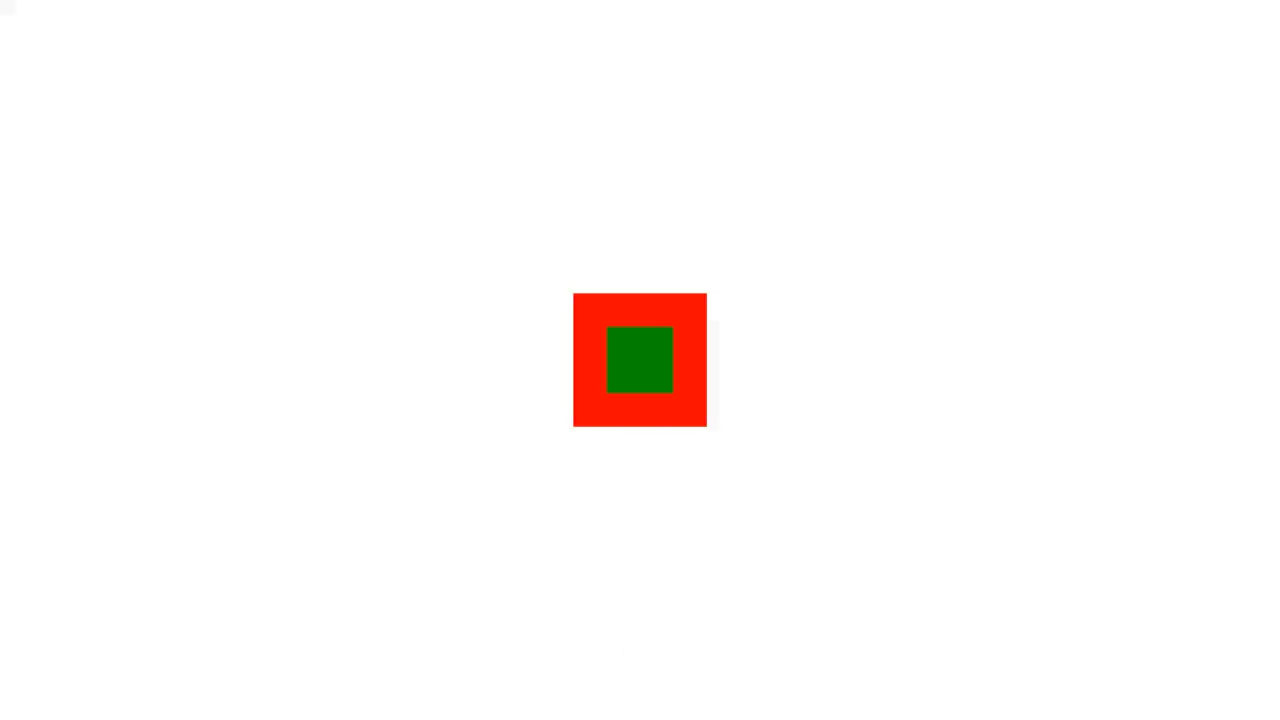
动画属性类型的值定义
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_ALPHA
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_ROTATION
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_ROTATION_X
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_ROTATION_Y
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_ROTATION_Z
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_SCALE_X
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_SCALE_Y
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_SCALE_Z
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_TRANSLATION_X
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_TRANSLATION_Y
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_TRANSLATION_Z
示例代码
animationViewRef.value?.objectAnimator2(
"2",//自定义id
QTAnimationValueType.QT_ANIMATION_VALUE_TYPE_FLOAT,
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_TRANSLATION_X,
0,
300,
1000,
-1,
0,
false,
false,
);
animationViewRef.value?.startAnimator("2");
动画合集
创建动画合集参数
animationRef.value?.animatorSet(
'SequentiallyAnimatorsId', //动画合集的id
-1, //动画合集执行的时长
false //是否监听动画合集执行状态
);
动画合集的id
动画合集通过自定义的动画id
执行所有的操作。
动画合集执行的时长
Sets the length of each of the current child animations of this AnimatorSet. By default, each child animation will use its own duration. If the duration is set on the AnimatorSet, then each child animation inherits this duration.
监听动画合集执行状态
顺序执行
效果预览
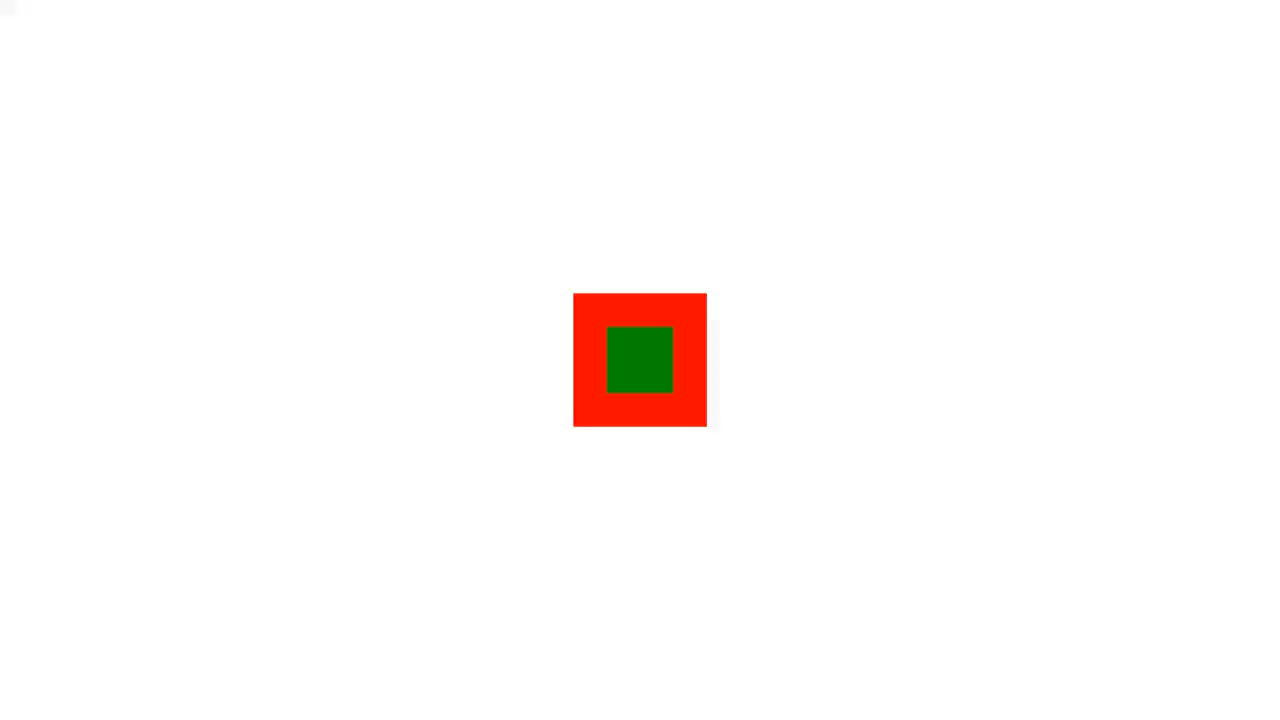
第1步:创建动画合集
//动画合集的id为:SequentiallyAnimatorsId
animationRef.value?.animatorSet('SequentiallyAnimatorsId', -1, false);
第2步:创建动画并加入到动画合集
animationRef.value?.objectAnimator2('AlphaAnimationId');
animationRef.value?.objectAnimator2('ScaleAnimationId');
animationRef.value?.objectAnimator2('RotationAnimationId');
animationRef.value?.objectAnimator2('TranslationAnimationId');
//playSequentially4
animationRef.value?.playSequentially4(
//动画合集的id
'SequentiallyAnimatorsId',
//动画的id
"AlphaAnimationId",
"ScaleAnimationId",
"RotationAnimationId",
"TranslationAnimationId",
);
第3步:执行动画合集
animationRef.value?.startAnimator('SequentiallyAnimatorsId');
并行执行
效果预览
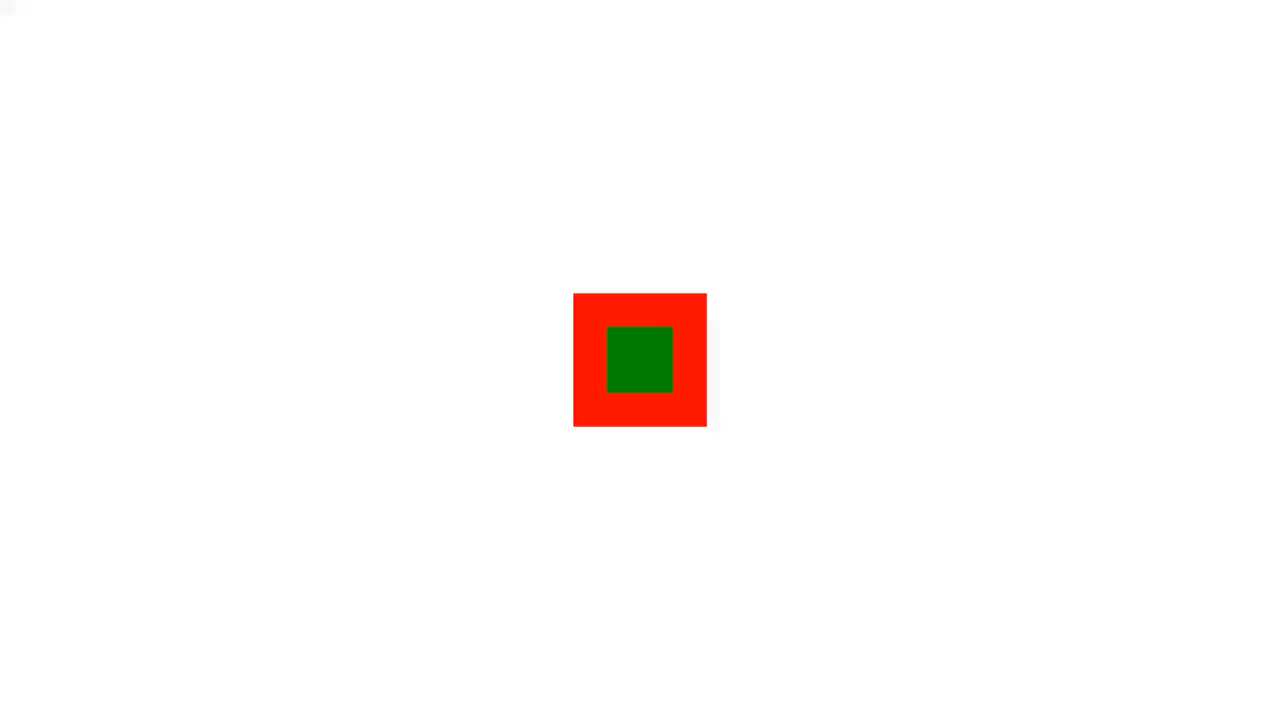
第1步:创建动画合集
//动画合集的id为:TogetherAnimatorsId
animationRef.value?.animatorSet('TogetherAnimatorsId', -1, false);
第2步:创建动画并加入到动画合集
animationRef.value?.objectAnimator2('AlphaAnimationId');
animationRef.value?.objectAnimator2('ScaleAnimationId');
animationRef.value?.objectAnimator2('RotationAnimationId');
animationRef.value?.objectAnimator2('TranslationAnimationId');
//playTogether4
animationRef.value?.playTogether4(
//动画合集的id
'TogetherAnimatorsId',
//动画的id
"AlphaAnimationId",
"ScaleAnimationId",
"RotationAnimationId",
"TranslationAnimationId",
);
第3步:执行动画合集
animationRef.value?.startAnimator('TogetherAnimatorsId');
with
效果预览
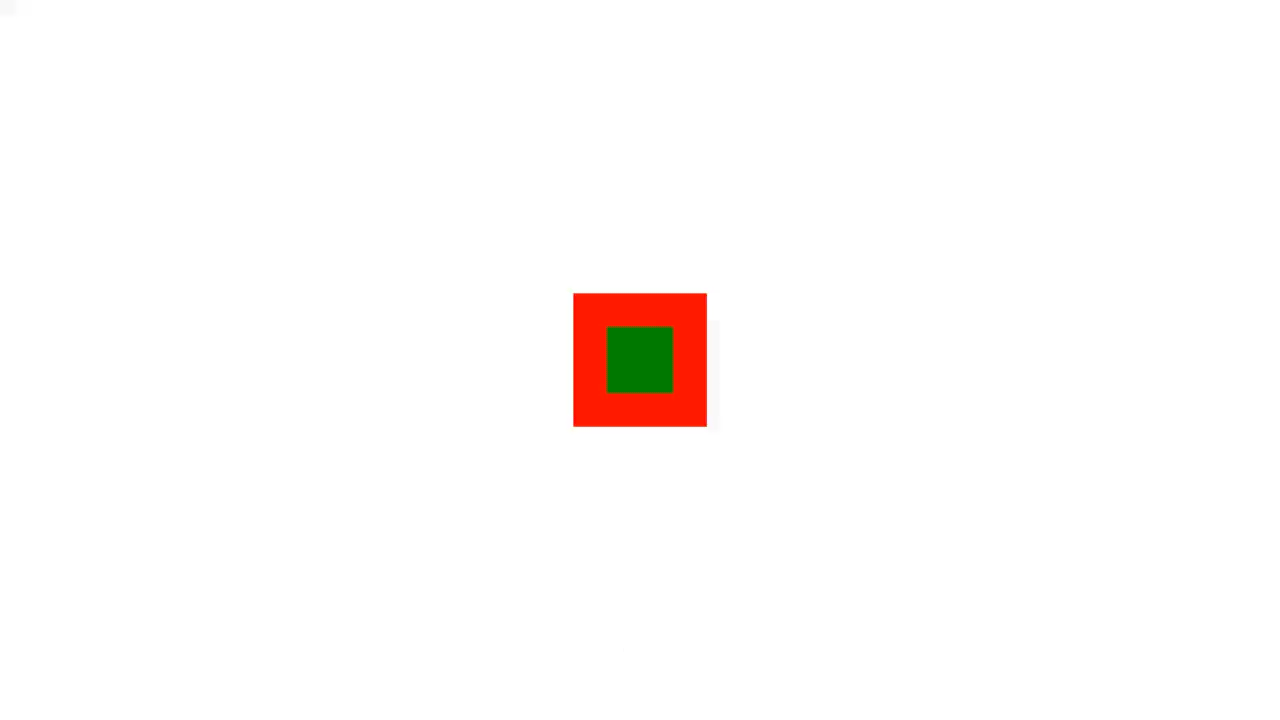
第1步:创建动画合集
//动画合集的id为:WithAnimatorsId
animationRef.value?.animatorSet('WithAnimatorsId', -1, false);
第2步:创建动画并加入到动画合集
animationRef.value?.objectAnimator2('AlphaAnimationId');
animationRef.value?.objectAnimator2('ScaleAnimationId');
//AlphaAnimationId
animationRef.value?.play(
'WithAnimatorsId',
"AlphaAnimationId",
);
//ScaleAnimationId
animationRef.value?.with(
'WithAnimatorsId',
"ScaleAnimationId",
);
第3步:执行动画合集
animationRef.value?.startAnimator('WithAnimatorsId');
before
效果预览
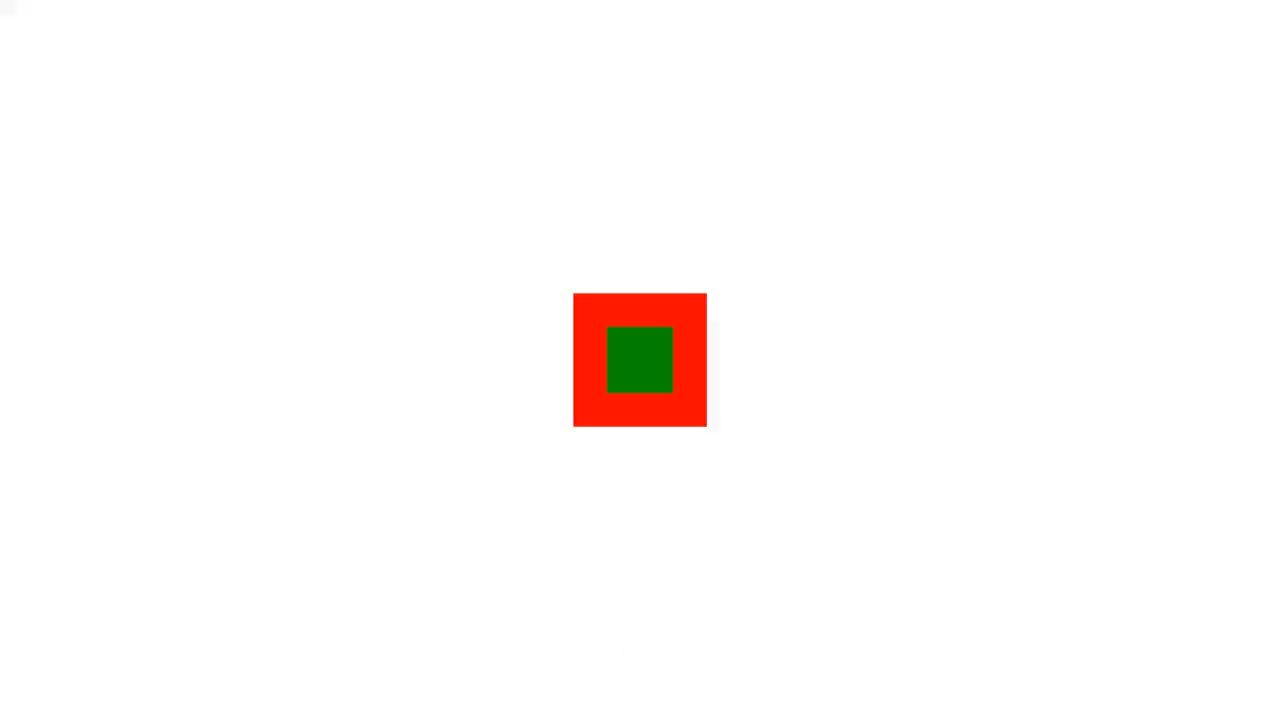
第1步:创建动画合集
//动画合集的id为:BeforeAnimatorsId
animationRef.value?.animatorSet('BeforeAnimatorsId', -1, false);
第2步:创建动画并加入到动画合集
animationRef.value?.objectAnimator2('AlphaAnimationId');
animationRef.value?.objectAnimator2('ScaleAnimationId');
//AlphaAnimationId
animationRef.value?.play(
'BeforeAnimatorsId',
"AlphaAnimationId",
);
//ScaleAnimationId
animationRef.value?.before(
'BeforeAnimatorsId',
"ScaleAnimationId",
);
第3步:执行动画合集
animationRef.value?.startAnimator('BeforeAnimatorsId');
after
效果预览
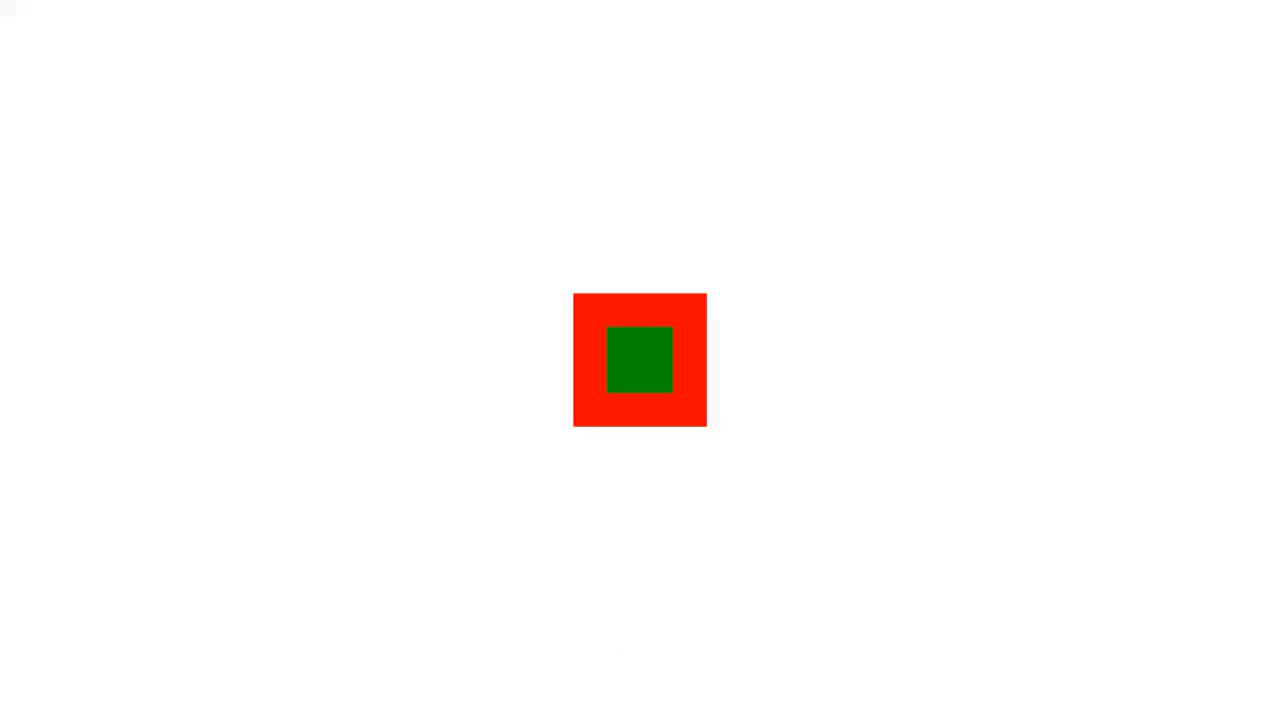
第1步:创建动画合集
//动画合集的id为:AfterAnimatorsId
animationRef.value?.animatorSet('AfterAnimatorsId', -1, false);
第2步:创建动画并加入到动画合集
animationRef.value?.objectAnimator2('AlphaAnimationId');
animationRef.value?.objectAnimator2('ScaleAnimationId');
//AlphaAnimationId
animationRef.value?.play(
'AfterAnimatorsId',
"AlphaAnimationId",
);
//ScaleAnimationId
animationRef.value?.after(
'AfterAnimatorsId',
"ScaleAnimationId",
);
第3步:执行动画合集
animationRef.value?.startAnimator('AfterAnimatorsId');
动画插值器
属性动画动画插值器分类
QTAnimationInterpolatorType.QT_ACCELERATE_DECELERATE_INTERPOLATOR
QTAnimationInterpolatorType.QT_ACCELERATE_INTERPOLATOR
QTAnimationInterpolatorType.QT_ANTICIPATE_INTERPOLATOR
QTAnimationInterpolatorType.QT_ANTICIPATE_OVERSHOOT_INTERPOLATOR
QTAnimationInterpolatorType.QT_BOUNCE_INTERPOLATOR
QTAnimationInterpolatorType.QT_CYCLE_INTERPOLATOR
QTAnimationInterpolatorType.QT_DECELERATE_INTERPOLATOR
QTAnimationInterpolatorType.QT_LINEAR_INTERPOLATOR
QTAnimationInterpolatorType.QT_OVERSHOOT_INTERPOLATOR
QTAnimationInterpolatorType.QT_FAST_OUT_LINEAR_IN_INTERPOLATOR
QTAnimationInterpolatorType.QT_FAST_OUT_SLOW_IN_INTERPOLATOR
QTAnimationInterpolatorType.QT_PATH_INTERPOLATOR
示例代码
animationRef.value?.objectAnimator3(
"AccelerateInterpolator",//自定义id
QTAnimationValueType.QT_ANIMATION_VALUE_TYPE_FLOAT,
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_ALPHA,
"0",
"500",
'0',
1500,
-1,
0,
false,
false,
//插值器参数对象
{
type: QTAnimationInterpolatorType.QT_LINEAR_INTERPOLATOR,
},
);
动画监听
监听动画执行状态
第1步:创建动画的时候需要设置是否监听动画状态变化
animationRef.value?.objectAnimator2(
"1",//自定义id
QTAnimationValueType.QT_ANIMATION_VALUE_TYPE_FLOAT,
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_TRANSLATION_X,
"0",
"1",
1000,
-1,
0,
true,//监听动画执行状态
false,
null,
);
第2步: <qt-animation>
标签上监听动画执行状态
<template>
<qt-animation
class="animation-view-css"
@onAnimationCancel="onAnimationCancel"
@onAnimationEnd="onAnimationEnd"
@onAnimationStart="onAnimationStart"
@onAnimationRepeat="onAnimationRepeat"
@onAnimationPause="onAnimationPause"
@onAnimationResume="onAnimationResume">
<div class="animation-inner-view-css" />
</qt-animation>
</template>
<script lang="ts">
import { defineComponent } from "@vue/runtime-core";
import { ESLogLevel, useESLog } from "@extscreen/es3-core";
const TAG = 'Animation'
export default defineComponent({
setup() {
const log = useESLog()
function onAnimationCancel(id) {
if (log.isLoggable(ESLogLevel.DEBUG)) {
log.d(TAG, '-------onAnimationCancel------->>>>', id)
}
}
function onAnimationEnd(id, isReverse) {
if (log.isLoggable(ESLogLevel.DEBUG)) {
log.d(TAG, '-------onAnimationEnd------->>>>', id, isReverse)
}
}
function onAnimationRepeat(id) {
if (log.isLoggable(ESLogLevel.DEBUG)) {
log.d(TAG, '-------onAnimationRepeat------->>>>', id)
}
}
function onAnimationStart(id, isReverse) {
if (log.isLoggable(ESLogLevel.DEBUG)) {
log.d(TAG, '-------onAnimationStart------->>>>', id, isReverse)
}
}
function onAnimationPause(id) {
if (log.isLoggable(ESLogLevel.DEBUG)) {
log.d(TAG, '-------onAnimationPause------->>>>', id)
}
}
function onAnimationResume(id) {
if (log.isLoggable(ESLogLevel.DEBUG)) {
log.d(TAG, '-------onAnimationResume------->>>>', id)
}
}
return {
onAnimationCancel,
onAnimationEnd,
onAnimationRepeat,
onAnimationStart,
onAnimationPause,
onAnimationResume,
}
},
});
</script>
onAnimationStart
:动画开始 onAnimationEnd
:动画结束 onAnimationCancel
:动画取消 onAnimationRepeat
:动画重复执行 onAnimationPause
:动画暂停 onAnimationResume
:动画恢复
监听动画属性值变化
第1步: 创建动画的时候需要设置是否监听动画属性值变化
animationViewRef.value?.objectAnimator2(
"1",//自定义id
QTAnimationValueType.QT_ANIMATION_VALUE_TYPE_FLOAT,
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_TRANSLATION_X,
"0",
"1",
1000,
-1,
0,
false,
true,//监听动画属性值变化
null,
);
第2步: <qt-animation>
标签上监听动画属性值变化
<template>
<qt-animation
class="animation-view-css"
@onAnimationUpdate="onAnimationUpdate">
<div class="animation-inner-view-css" />
</qt-animation>
</template>
<script lang="ts">
import { defineComponent } from "@vue/runtime-core";
import { ESLogLevel, useESLog } from "@extscreen/es3-core";
const TAG = 'Animation'
export default defineComponent({
setup() {
const log = useESLog()
function onAnimationUpdate(id, value) {
if (log.isLoggable(ESLogLevel.DEBUG)) {
log.d(TAG, '-------onAnimationUpdate------->>>>', id, value)
}
}
return {
onAnimationUpdate,
}
},
});
</script>
onAnimationUpdate(id, value)
:id
为动画的id,value
为动画当前时刻的属性值
动画重复模式
属性动画重复模式
Defines what this animation should do when it reaches the end. This setting is applied only when the repeat count is either greater than 0 or INFINITE. Defaults to RESTART.
restart
reverse
infinite
属性动画重复模式的值定义:
QTAnimationRepeatMode.QT_ANIMATION_REPEAT_MODE_RESTART
QTAnimationRepeatMode.QT_ANIMATION_REPEAT_MODE_REVERSE
QTAnimationRepeatMode.QT_ANIMATION_REPEAT_MODE_INFINITE
属性动画重复次数:
Sets how many times the animation should be repeated. If the repeat count is 0, the animation is never repeated. If the repeat count is greater than 0 or INFINITE, the repeat mode will be taken into account. The repeat count is 0 by default.
示例代码
animationViewRef.value?.objectAnimator5(
"2",//自定义id
QTAnimationValueType.QT_ANIMATION_VALUE_TYPE_FLOAT,
QTAnimationPropertyName.QT_ANIMATION_PROPERTY_NAME_TRANSLATION_Y,
0,
300,
0,
-300,
0,
1000,
QTAnimationRepeatMode.QT_ANIMATION_REPEAT_MODE_REVERSE,
2,
false,
false,
);
API
Events
Name | Description | Type |
---|---|---|
onAnimationEnd | 动画结束事件 | Function |
onAnimationCancel | 动画取消事件 | Function |
onAnimationStart | 动画开始事件 | Function |
onAnimationRepeat | 动画重复执行事件 | Function |
onAnimationPause | 动画暂停事件 | Function |
onAnimationResume | 动画恢复事件 | Function |
onAnimationUpdate | 动画更新事件 | Function |
Slots
Name | Description |
---|---|
default | 需要执行动画的组件 |
Exposes
Name | Description | Type |
---|---|---|
setPivotX | 设置x轴方向的旋转点 | Function |
setPivotY | 设置y轴方向的旋转点 | Function |
resetPivot | 重置旋转点 | Function |
resetAnimators | 重置所有动画 | Function |
animatorSet | 创建动画集合 | Function |
startAnimator | 开始动画 | Function |
startAnimatorDelay | 延迟开始动画 | Function |
pauseAnimator | 暂停动画 | Function |
resumeAnimator | 恢复动画 | Function |
cancelAnimator | 取消动画 | Function |
reverseAnimator | 逆转动画 | Function |
play | 播放动画 | Function |
playWith | 同时播放动画 | Function |
playBefore | 在之前播放动画 | Function |
playAfter | 在之后播放动画 | Function |
playAfterDelay | 在之后延迟播放动画 | Function |
playSequentially1 | 顺序播放动画 | Function |
playSequentially2 | 顺序播放动画 | Function |
playSequentially3 | 顺序播放动画 | Function |
playSequentially4 | 顺序播放动画 | Function |
playSequentially5 | 顺序播放动画 | Function |
playTogether1 | 同时播放动画 | Function |
playTogether2 | 同时播放动画 | Function |
playTogether3 | 同时播放动画 | Function |
playTogether4 | 同时播放动画 | Function |
playTogether5 | 同时播放动画 | Function |
objectAnimator1 | 创建动画 | Function |
objectAnimator2 | 创建动画 | Function |
objectAnimator3 | 创建动画 | Function |
objectAnimator4 | 创建动画 | Function |
objectAnimator5 | 创建动画 | Function |
objectAnimator6 | 创建动画 | Function |
objectAnimator7 | 创建动画 | Function |
objectAnimator8 | 创建动画 | Function |
objectAnimator9 | 创建动画 | Function |
objectAnimator10 | 创建动画 | Function |